|
|
連載:XAMLの基礎知識
第2回 XAMLとWPFの関係
デジタルアドバンテージ 遠藤 孝信
2008/02/15 |
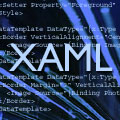 |
|
ウィンドウをXMALではなくC#/VBで記述
いまイベント・ハンドラだけをC#/VBで記述しましたが、続いてはウィンドウの定義であるwin.xamlの内容をすべてC#/VBで記述してみましょう。
といってもそれほど難しくなく、先ほどイベント・ハンドラを記述したリスト5にHelloWorldWindowクラスのコンストラクタを追加し、そこでウィンドウの初期設定を行うだけです。具体的には、ウィンドウのプロパティ設定と、ボタンの作成/追加を行います。このファイル名はwin.cs/win.vbとします。
using System.Windows;
using System.Windows.Controls;
public class HelloWorldWindow : Window {
public HelloWorldWindow() { // コンストラクタ
this.Title = "My First XAML";
this.Width = 320;
this.Height = 240;
Button button = new Button();
button.Content = "Click Me!"; // ボタンのキャプションを設定
button.Width = 100;
button.Height = 50;
button.Click += HelloWorldWindow_Click;
this.Content = button; // ボタンをウィンドウに追加
}
void HelloWorldWindow_Click(object sender, RoutedEventArgs e) {
MessageBox.Show("クリックされました!");
}
}
|
Imports System.Windows
Imports System.Windows.Controls
Partial Public Class HelloWorldWindow
Inherits Window
Public Sub New() ' コンストラクタ
Me.Title = "My First XAML"
Me.Width = 320
Me.Height = 240
Dim button As New Button()
button.Content = " Click Me!" ' ボタンのキャプションを設定
button.Width = 100
button.Height = 50
AddHandler button.Click, AddressOf HelloWorldWindow_Click
Me.Content = button ' ボタンをウィンドウに追加
End Sub
Sub HelloWorldWindow_Click(ByVal sender As Object, ByVal e As RoutedEventArgs)
MessageBox.Show("クリックされました!")
End Sub
End Class
|
|
リスト7 win.xamlの内容をC#/VBで記述したコード(上:win.cs、下:win.vb) |
XAMLで<Window>要素の属性として記述していた「Title」や「Width」「Height」などは、Windowクラスのプロパティとして記述します。また、<Button>要素のClick属性は、ButtonクラスではClickイベントに対応しています。このリスト7の構造は従来のWindowsフォームでのウィンドウとほぼ同じといえるでしょう。
ただしContentプロパティは少しだけ特殊で、これにはまさにコントロールのコンテンツ(=内容)となるものを指定します。XAML上では<Window>タグと</Window>タグ、<Button>タグと</Button>タグで囲まれていた内容が、実はContentプロパティの内容となります。コンテンツがコントロールのどの部分の内容になるかはコントロールによって異なります。
なお、もはや部分クラスを使う必要はありませんので、リスト7ではpartialキーワードを削除しています。
アプリケーション定義におけるメソッド呼び出し
いま作成したリスト7だけでは、まだビルドはできません。続いては、リスト7で記述したHelloWorldWindowクラスのインスタンス化について考える必要があります。
ここまでは、リスト2に示したアプリケーションの定義において、<Application>要素のStartupUri属性にwin.xamlを指定することにより、ウィンドウのインスタンス化が可能でした。しかし、ここではもはやwin.xamlは使用しませんので、HelloWorldWindowクラスを“New”するコードを実行する必要があります。
<Application>要素に対応するSystem.Windows名前空間のApplicationクラスには、アプリケーション開始時に発生する「Startupイベント」が用意されているため、これを使いましょう。つまり、先ほどのボタンのClickイベントと同様の手法により、Startupイベントのイベント・ハンドラをC#/VBで記述し、そこでウィンドウのインスタンス化を行います。
まず、<Application>要素に対しては、x:Class属性でアプリケーションのクラス名を指定し、Startup属性にイベント・ハンドラとなるメソッド名を指定します。
<Application
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="HelloWorldApp"
Startup="HelloWorldApp_Startup"
/>
|
|
リスト8 Startupイベントを追加したapp.xaml |
このXAMLファイルのコードビハインド・ファイルは次のようになります。HelloWorldWindowクラスをインスタンス化し、そのShowメソッドによりウィンドウを表示するだけです。
using System.Windows;
public partial class HelloWorldApp : Application
{
void HelloWorldApp_Startup(object sender, StartupEventArgs e)
{
HelloWorldWindow win = new HelloWorldWindow();
win.Show();
}
}
|
Imports System.Windows
Partial Public Class HelloWorldApp
Inherits Application
Sub HelloWorldApp_Startup(ByVal sender As Object, ByVal e As StartupEventArgs)
Dim win As New HelloWorldWindow()
win.Show()
End Sub
End Class
|
|
リスト9 Startupイベントを追加したapp.xamlのコードビハインド・ファイル(上:app.xaml.cs、下:app.xaml.vb) |
そして、リスト7〜9をビルドするためのプロジェクト・ファイルは次のようになります。
<Project
xmlns="http://schemas.microsoft.com/developer/msbuild/2003"
DefaultTargets="Build" >
<PropertyGroup>
<AssemblyName>HelloWorld</AssemblyName>
<OutputType>winexe</OutputType>
<OutputPath>.\</OutputPath>
</PropertyGroup>
<ItemGroup>
<ApplicationDefinition Include="app.xaml" />
<Compile Include="app.xaml.cs" />
<Compile Include="win.cs" />
<Reference Include="System" />
<Reference Include="WindowsBase" />
<Reference Include="PresentationCore" />
<Reference Include="PresentationFramework" />
</ItemGroup>
<Import Project="$(MSBuildBinPath)\Microsoft.CSharp.targets" />
<Import Project="$(MSBuildBinPath)\Microsoft.WinFX.targets" />
</Project>
|
<Project
xmlns="http://schemas.microsoft.com/developer/msbuild/2003"
DefaultTargets="Build" >
<PropertyGroup>
<AssemblyName>HelloWorld</AssemblyName>
<OutputType>winexe</OutputType>
<OutputPath>.\</OutputPath>
</PropertyGroup>
<ItemGroup>
<ApplicationDefinition Include="app.xaml" />
<Compile Include="app.xaml.vb" />
<Compile Include="win.vb" />
<Reference Include="System" />
<Reference Include="WindowsBase" />
<Reference Include="PresentationCore" />
<Reference Include="PresentationFramework" />
</ItemGroup>
<Import Project="$(MSBuildBinPath)\Microsoft.VisualBasic.targets" />
<Import Project="$(MSBuildBinPath)\Microsoft.WinFX.targets" />
</Project>
|
|
リスト10 プロジェクト・ファイルhello.proj(上:C#用、下:VB用) |
リスト7〜9からHelloWorld.exeを作成するためのプロジェクト・ファイル。 |
 |
 |
Insider.NET 記事ランキング
本日
月間