|
|
特集:VBでOracle Database開発入門(後編)
SQL Server開発者のためのOracle DB入門
初音 玲
2008/12/09 |
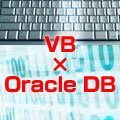 |
|
○データの取得(DataReaderクラス)
画面に表示しないデータを取得する場合は、DataReaderクラスを使用するのが一般的だ。SQL ServerとOracle Databaseでは、SqlDataReaderクラス(System.Data.SqlClient名前空間)とOracleDataReaderクラス(Oracle.DataAccess.Client名前空間)というようにDataReaderクラスの名前に多少の相違はあるが、メンバ(プロパティ、メソッド、イベント)名や使い方は両者で違いはほとんどない。
次のリストは、実際にデータを取得する両者のサンプル・コードだ。employee(SQL Server)/EMP(Oracle Database)テーブルの内容を取得し、そのデータのfname(SQL Server)/ENAME(Oracle Database)フィールドの値を1件ずつListBox1コントロールに追加する処理を実装している。
Imports System.Data.SqlClient
Public Class DataReader
Private Const CnString As String = _
"User Id=sa;" & _
"Password=;" & _
"Initial Catalog=pubs;" & _
"Data Source=localhost\SQLEXPRESS;"
Private Const SqlString As String = "SELECT * FROM employee"
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Using _cn As New SqlConnection(CnString)
_cn.Open()
Using _cmd As New SqlCommand
Dim rd As SqlDataReader = Nothing
_cmd.Connection = _cn
_cmd.CommandText = SqlString
Try
Me.ListBox1.Items.Clear()
rd = _cmd.ExecuteReader
Do While rd.Read
Me.ListBox1.Items.Add(rd.Item("fname").ToString)
Loop
Catch ex As Exception
MessageBox.Show(ex.Message, _
Me.Text,_
MessageBoxButtons.OK,_
MessageBoxIcon.Exclamation)
Finally
If Not rd Is Nothing Then
rd.Close()
End If
End Try
End Using
_cn.Close()
End Using
End Sub
End Class |
Imports Oracle.DataAccess.Client
Public Class DataReader
Private Const CnString As String = _
"User Id=scott;" & _
"Password=tiger;" & _
"Data Source=192.168.11.10:1521/orcl.ora11g.hogehoge.com;"
Private Const SqlString As String = "SELECT * FROM EMP"
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Using _cn As New OracleConnection(CnString)
_cn.Open()
Using _cmd As New OracleCommand
Dim rd As OracleDataReader = Nothing
_cmd.Connection = _cn
_cmd.CommandText = SqlString
Try
Me.ListBox1.Items.Clear()
rd = _cmd.ExecuteReader
Do While rd.Read
Me.ListBox1.Items.Add(rd.Item("ENAME").ToString)
Loop
Catch ex As Exception
MessageBox.Show(ex.Message, _
Me.Text, _
MessageBoxButtons.OK, _
MessageBoxIcon.Exclamation)
Finally
If Not rd Is Nothing Then
rd.Close()
End If
End Try
End Using
_cn.Close()
End Using
End Sub
End Class |
|
DataReaderクラスによりデータを取得するコード(上:SQL Server、下:Oracle Database) |
○データの取得と更新(データアダプタ)
画面に表示するデータを取得する場合や、そのデータをユーザーが変更し、それをデータベースに反映したい場合には、データセット(DataSetクラス)を使用するのが一般的だ。データセットは、すべての.NETデータ・プロバイダに共通で利用できるので、データセットによりデータを扱う場合にはSQL ServerとOracle Database間での違いを考慮をする必要はない。
データセットは、そのデータソースとして.NETデータ・プロバイダに含まれるデータアダプタ(DataAdapterクラス)やコマンド(Commandクラス)を利用する。この.NETデータ・プロバイダとデータセットの関係を示したのが次の図である。
 |
図4 .NETデータ・プロバイダとデータセットの関係 |
アプリケーション内のコードで、Commandクラスやデータアダプタ、データセットのインスタンスを生成し、データセットを活用することでデータの取得と更新を行う。データセットは、生成しておいたデータアダプタを通じてデータソースと結び付けられる。 |
.NETデータ・プロバイダの各クラスは、データベースごとに異なる。SQL ServerのデータアダプタはSqlDataAdapterクラス、コマンドはSqlCommandクラス、そしてOracle DatabaseのデータアダプタはOracleDataAdapterクラス、コマンドはOracleCommandクラスである。
以下のコードは、SQL ServerとOracle Databaseのそれぞれでデータアダプタとデータセットを活用した例だ。実装内容は、Button1コントロールをクリックすると、employee/EMPテーブルのデータがDataGridView1コントロールに表示される。また、Button2コントロールをクリックすると、employee/EMPテーブルのデータを更新するが、こちらはトランザクション処理も同時に実装されている。
Imports System.Data.SqlClient
Public Class DataAdapter
Private Const CnString As String = _
"User Id=sa;" & _
"Password=;" & _
"Initial Catalog=pubs;" & _
"Data Source=localhost\SQLEXPRESS;"
Private Ds As New DataSet
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Const sqlString As String = "SELECT * FROM employee "
Using _cn As New SqlConnection(CnString)
_cn.Open()
Using _cmd As New SqlCommand(sqlString, _cn)
Using _da As New SqlDataAdapter(_cmd)
Try
_da.Fill(Ds, "employee")
MessageBox.Show("Fill", Me.Text)
Me.DataGridView1.DataSource = Ds.Tables("employee")
Catch ex As Exception
MessageBox.Show(ex.Message, _
Me.Text, _
MessageBoxButtons.OK, _
MessageBoxIcon.Exclamation)
Finally
End Try
End Using
End Using
_cn.Close()
End Using
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
Const sqlString As String = "SELECT * FROM employee "
Using _cn As New SqlConnection(CnString)
_cn.Open()
Using _tr As SqlTransaction = _cn.BeginTransaction()
Using _cmd As New SqlCommand(sqlString, _cn)
_cmd.Transaction = _tr '###重要###
Using _da As New SqlDataAdapter(_cmd)
Using cb As New SqlCommandBuilder(_da)
_da.UpdateCommand = cb.GetUpdateCommand()
_da.InsertCommand = cb.GetInsertCommand()
_da.DeleteCommand = cb.GetDeleteCommand()
MessageBox.Show(_da.UpdateCommand.CommandText)
MessageBox.Show(_da.InsertCommand.CommandText)
MessageBox.Show(_da.DeleteCommand.CommandText)
Dim isOK As Boolean = False
Try
_da.Update(Ds, "employee")
MessageBox.Show("Update", Me.Text)
isOK = True
Catch ex As Exception
MessageBox.Show(ex.Message, _
Me.Text, _
MessageBoxButtons.OK, _
MessageBoxIcon.Exclamation)
Finally
If isOK Then
_tr.Commit()
Else
_tr.Rollback()
End If
End Try
End Using
End Using
End Using
End Using
End Using
End Sub
End Class |
Imports Oracle.DataAccess.Client
Public Class DataAdapter
Private Const CnString As String = _
"User Id=scott;" & _
"Password=tiger;" & _
"Data Source=192.168.11.10:1521/orcl.ora11g.hogehoge.com;"
Private Ds As New DataSet
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Const sqlString As String = "SELECT * FROM EMP"
Using _cn As New OracleConnection(CnString)
_cn.Open()
Using _cmd As New OracleCommand(sqlString, _cn)
Using _da As New OracleDataAdapter(_cmd)
Try
_da.Fill(Ds, "EMP")
MessageBox.Show("Fill", Me.Text)
Me.DataGridView1.DataSource = Ds.Tables("EMP")
Catch ex As Exception
MessageBox.Show(ex.Message, _
Me.Text, _
MessageBoxButtons.OK, _
MessageBoxIcon.Exclamation)
Finally
End Try
End Using
End Using
_cn.Close()
End Using
End Sub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
Const sqlString As String = "SELECT * FROM EMP"
Using _cn As New OracleConnection(CnString)
_cn.Open()
Using _tr As OracleTransaction = _cn.BeginTransaction()
Using _cmd As New OracleCommand(sqlString, _cn)
_cmd.Transaction = _tr '###重要###
Using _da As New OracleDataAdapter(_cmd)
Using cb As New OracleCommandBuilder(_da)
_da.UpdateCommand = cb.GetUpdateCommand()
_da.InsertCommand = cb.GetInsertCommand()
_da.DeleteCommand = cb.GetDeleteCommand()
MessageBox.Show(_da.UpdateCommand.CommandText)
MessageBox.Show(_da.InsertCommand.CommandText)
MessageBox.Show(_da.DeleteCommand.CommandText)
Dim isOK As Boolean = False
Try
_da.Update(Ds, "EMP")
MessageBox.Show("Update", Me.Text)
isOK = True
Catch ex As Exception
MessageBox.Show(ex.Message, _
Me.Text, _
MessageBoxButtons.OK, _
MessageBoxIcon.Exclamation)
Finally
If isOK Then
_tr.Commit()
Else
_tr.Rollback()
End If
End Try
End Using
End Using
End Using
End Using
_cn.Close()
End Using
End Sub
End Class |
|
データアダプタとデータセットの活用例(上:SQL Server、下:Oracle Database) |
SQL Server開発者がOracle Database開発を始めるときには、以上で説明したOracle DatabaseとSQL Serverの差異を特に注意してほしい。
それでは次に、従来のVB6(Visual Basic 6)から.NETへ移行するときに、Oracle Database開発で特に注意すべきポイントについて説明しよう。
業務アプリInsider 記事ランキング
本日
月間