連載:改造WebアプリケーションUIビフォー/アフター
第1回 ASP.NETによる3階層Webアプリ「ITブック」構築
葛西秋雄
2010/02/05 |
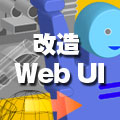 |
|
データアクセス層
「ITブック」のデータアクセス層は、ProductManagerDBクラスで構成されます。このクラスはビジネスロジック層にデータを渡すために、Productクラスを利用します。
Productクラスはエンティティ・クラスであり、XMLファイルから取得した商品データを格納するためのものです。Productクラスは、本来ビジネスロジック層に帰属するものですが、便宜的にここで掲載しています。
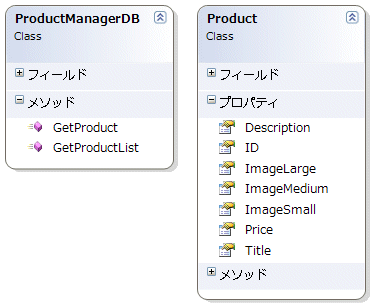
|
図2 データアクセス層に登録されているクラスとメソッド/プロパティ |
|
ProductManagerDBクラスのGetProductListメソッドは、App_Dataフォルダに格納されているXMLファイル(Books.xml)からすべての商品データを取得し、Productクラスに格納してコレクションを返します。
また、GetProductメソッドは、Books.xmlファイルから特定(ID)の商品データを検索して、Productクラスに格納して返します。GetProductメソッドの引数には、商品のIDを指定します。
以下にGetProductメソッドの内容を掲載します。
Public Shared Function GetProduct(ByVal intProductID As Integer) As Product
Dim ds As New DataSet()
Dim dt As DataTable
Dim clsProduct As New Product()
' DataTableがキャッシュされているか?
If HttpContext.Current.Cache(productList) IsNot Nothing Then
' キャッシュからDataTableを生成する
dt = CType(HttpContext.Current.Cache(productList), DataTable)
Else
' Books.xmlファイルを読み込んでDataTableに格納する
ds.ReadXml( _
HttpContext.Current.Server.MapPath("~\App_Data\books.xml"))
dt = ds.Tables(0)
' DataTableにキャッシュする
HttpContext.Current.Cache.Insert( _
productList, dt, Nothing, _
DateTime.Now.AddMinutes(20), TimeSpan.Zero)
End If
Dim dv As DataView = dt.DefaultView
' 指定したレコードを絞り込む
dv.RowFilter = "ID=" & intProductID
' 先頭レコードを取得する
Dim drv As DataRowView = dv(0)
' Productクラスに格納して返す
clsProduct.ID = drv("id")
clsProduct.Title = drv("title")
clsProduct.Description = drv("description")
clsProduct.Price = drv("price")
clsProduct.ImageLarge = drv("imageLarge")
clsProduct.ImageMedium = drv("imageMedium")
clsProduct.ImageSmall = drv("imageSmall")
Return clsProduct
End Function
|
public static Product GetProduct(int intProductID) {
DataSet ds = new DataSet();
DataTable dt = default(DataTable);
Product clsProduct = new Product();
// DataTableがキャッシュされているか?
if (HttpContext.Current.Cache[productList] != null) {
// キャッシュからDataTableを生成する
dt = (DataTable)HttpContext.Current.Cache[productList];
} else {
// Books.xmlファイルを読み込んでDataTableに格納する
ds.ReadXml(
HttpContext.Current.Server.MapPath("~\\App_Data\\books.xml"));
dt = ds.Tables[0];
// DataTableをキャッシュする
HttpContext.Current.Cache.Insert(
productList, dt, null,
DateTime.Now.AddMinutes(20), TimeSpan.Zero);
}
DataView dv = dt.DefaultView;
// 指定したレコードを絞り込む
dv.RowFilter = "ID=" + intProductID;
// 先頭レコードを取得する
DataRowView drv = dv[0];
// レコードをProductクラスに格納して返す
clsProduct.ID = int.Parse(drv["id"].ToString());
clsProduct.Title = drv["title"].ToString();
clsProduct.Description = drv["description"].ToString();
clsProduct.Price = decimal.Parse(drv["price"].ToString());
clsProduct.ImageLarge = drv["imageLarge"].ToString();
clsProduct.ImageMedium = drv["imageMedium"].ToString();
clsProduct.ImageSmall = drv["imageSmall"].ToString();
return clsProduct;
}
|
|
GetProductメソッドの内容(上:VB、下:C#) |
GetProductメソッドでは、App_Dataフォルダに格納されているBooks.xmlファイルをDataSetオブジェクトのReadXmlメソッドで読み込みます。そして、DataSetのTablesコレクションからDataTableを生成します。DataTableは、CacheオブジェクトのInsertメソッドにより、Webサーバのメモリ上に20分間キャッシュされます。
DataTableからDataViewを生成したら、RowFilterプロパティにフィルタ条件を設定して、商品データを絞り込みます。そして、DataViewから先頭のDataRowViewを取得して、Productクラスに格納して返します。
Books.xmlファイルには、次のような書籍データが格納されています。<id>要素には書籍のID、<title>要素には書籍のタイトル、<description>要素には書籍の説明文、<price>要素には書籍の定価が定義されています。<imagelarge>、<imagemedium>、<imagesmall>要素には、書籍の大、中、小のイメージのパスが定義されています。
<?xml version="1.0" encoding="utf-8" ?>
<books>
<book>
<id>5</id>
<title>ASP.NET 3.5 + jQuery Ajax 実践サンプル集</title>
<description>
……書籍の詳細……
</description>
<price>3800</price>
<imagelarge>books/images/asp.netjQueryLarge.jpg</imagelarge>
<imagemedium>books/images/asp.netjQueryMedium.jpg</imagemedium>
<imagesmall>books/images/asp.netjQuerySmall.jpg</imagesmall>
</book>
</books>
|
|
Books.xmlファイルの内容 |
ビジネスロジック層
ビジネスロジック層は、ShopManager、ShoppingCart、ProductManagerクラスから構成されます。OrderedProductは、エンティティ・クラスです。
ProductManagerクラスのGetProductList、GetProductメソッドは、データアクセス層のProductManagerDB.GetProductList、ProductManagerDB.GetProductメソッドを呼び出して商品データを要求します。
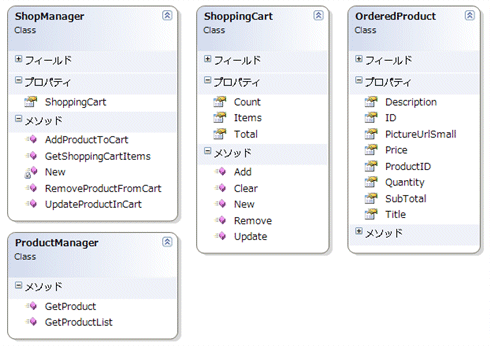
|
図3 ビジネスロジック層に登録されているクラスとメソッド/プロパティ |
|
ShopManagerクラスのGetShoppingCartItemsメソッドは、ショッピング・カート(ShoppingCart)に格納されているすべての商品(OrderedProduct)を取得して返します。
AddProductToCart、UpdateProductInCart、RemvoeProductFromCartメソッドは、ショッピング・カート(ShoppingCart)に商品(OrderedProduct)を追加したり、商品を更新、削除したりします。OrderedProductクラスには注文した商品データを格納します。ShoppingCartクラスには、OrderedProductクラスのコレクションが格納されます。
ここでは、便宜的にショッピング・カート(ShoppingCart)をASP.NETのセッション変数に格納していますが、本番で利用するときはプロファイルやデータベースに格納します。
' ショッピング・カートを生成してセッション変数に保存する
Public Shared ReadOnly Property ShoppingCart() As ShoppingCart
Get
' ショッピング・カートがセッションに存在しない?
If HttpContext.Current.Session(shoppintCart) Is Nothing Then
' 空のショッピング・カートを生成してセッションに保存する
HttpContext.Current.Session(shoppintCart) = New ShoppingCart()
End If
' セッションからショッピング・カートを生成して返す
Return CType(HttpContext.Current.Session(shoppintCart), ShoppingCart)
End Get
End Property
' ショッピング・カートに格納されている商品を取得する
Public Shared Function GetShoppingCartItems() As List(Of OrderedProduct)
Return ShoppingCart.Items
End Function
' 選択した商品をショッピング・カートに追加する
Public Shared Sub AddProductToCart(ByVal clsProduct As Product)
ShopManager.ShoppingCart.Add(clsProduct)
End Sub
' ショッピング・カートに格納されている商品の数量を更新する
Public Shared Sub UpdateProductInCart(ByVal newQuantity As Integer, ByVal ID As Guid)
ShoppingCart.Update(newQuantity, ID)
End Sub
' ショッピング・カートから特定の商品を削除する
Public Shared Sub RemoveProductFromCart(ByVal ID As Guid)
ShoppingCart.Remove(ID)
End Sub
|
// ショッピング・カートを生成してセッション変数に保存する
public static ShoppingCart ShoppingCart {
get {
// ショッピング・カートがセッションに存在しない?
if (HttpContext.Current.Session[shoppintCart] == null) {
// 空のショッピング・カートを生成してセッションに保存する
HttpContext.Current.Session[shoppintCart] = new ShoppingCart();
}
// セッションからショッピング・カートを生成して返す
return (ShoppingCart)HttpContext.Current.Session[shoppintCart];
}
}
// ショッピング・カートに格納されている商品を取得する
public static List<OrderedProduct> GetShoppingCartItems() {
return ShoppingCart.Items;
}
// 選択した商品をショッピング・カートに追加する
public static void AddProductToCart(Product clsProduct) {
ShopManager.ShoppingCart.Add(clsProduct);
}
// ショッピング・カートに格納されている商品の数量を更新する
public static void UpdateProductInCart(int newQuantity, Guid ID) {
ShoppingCart.Update(newQuantity, ID);
}
// ショッピング・カートから特定の商品を削除する
public static void RemoveProductFromCart(Guid ID) {
ShoppingCart.Remove(ID);
}
|
|
ShopManagerクラスの内容(上:VB、下:C#) |
 |
 |
業務アプリInsider 記事ランキング
本日
月間